AWS Lambda - JavaScript
Pre-requisites
- First Orion Branded Communications agreement
- Access to First Orion Customer Portal
- Vetted and Approved Business
- Ability to originate phone calls from configured phone numbers in calling platform
- Understanding of current calling platform and environment to integrate required API
Generate First Orion API Keys
Check out this guide for API Credentials - Guide Link
Lambda Setup
Lambda Directory and Code
- Create a local directory with this index.mjs file inside. Later, this will be the Lambda zip folder that's uploaded.
- If needed, change the a_number and b_number variables in the code to correspond to the payload from your input source.
- This example is being used by Amazon Connect. Connect documentation: doc link
// index.mjs
import axios from 'axios';
export const handler = async (event) => {
var aNumber = event.Details.ContactData.SystemEndpoint.Address // Phone number making the call.
var bNumber = event.Details.ContactData.CustomerEndpoint.Address // Phone number being called.
// For your logging processes.
console.log("aNumber: " + aNumber);
// Makes the pre-call push.
var precall = await pushPrecall(aNumber,bNumber);
console.log("Pre-call Status: " +precall.statusCode)
console.log("Pre-call Message: " +precall.body)
return precall;
};
const getToken = async () => {
var apiKey = process.env.API_KEY;
var secretKey = process.env.SECRET_KEY;
try {
const response = await axios.post(
'https://api.firstorion.com/v1/auth',
null, // No request body data
{
headers: {
'X-SERVICE':'auth',
'X-API-KEY': apiKey,
'X-SECRET-KEY': secretKey,
'Content-Type': 'application/json'
}
}
);
return response.data.token;
} catch (error) {
console.error('Error:', error);
return {
statusCode: error.response ? error.response.status : 500,
body: JSON.stringify({ error: error.message })
};
}
}
const pushPrecall = async (aNumber,bNumber) => {
var token = await getToken();
// console.log(token);
try{
const response = await axios.post('https://api.firstorion.com/exchange/v1/calls/push',
{
"aNumber": aNumber,
"bNumber": bNumber
}, {
headers: {
'Authorization': token,
'content-type': 'application/json'
}
});
// console.log(response.data)
// return(response.status)
const request = {
statusCode: response.status,
body: JSON.stringify(response.data.body.message),
};
return request;
}
catch (error) {
const request = {
statusCode: 500,
body: JSON.stringify(error),
};
return request;
}
}
// index.mjs
...
export const handler = async (event) => {
var a_number = "+15555555555" // Phone number making the call.
var b_number = "+15554444444"
...
Lambda Code Libraries
This Lambda requires the axios library to be included in the Lambda directory. Here is a method to include that library.
-
Navigate to the directory containing the index.mjs file.
-
Open this directory in a terminal and run the following. This should create the necessary library folders.
npm install axios
-
Compress/ZIP those folders with the lambda code from inside the folder. This will be uploaded to the Lambda
Lambda Creation
1. Create the Lambda
Environment Information
- Function Runtime: NodeJS 20.x
- Architecture: x86_64
2. Upload Lambda Zip
- This is the compressed folder from above
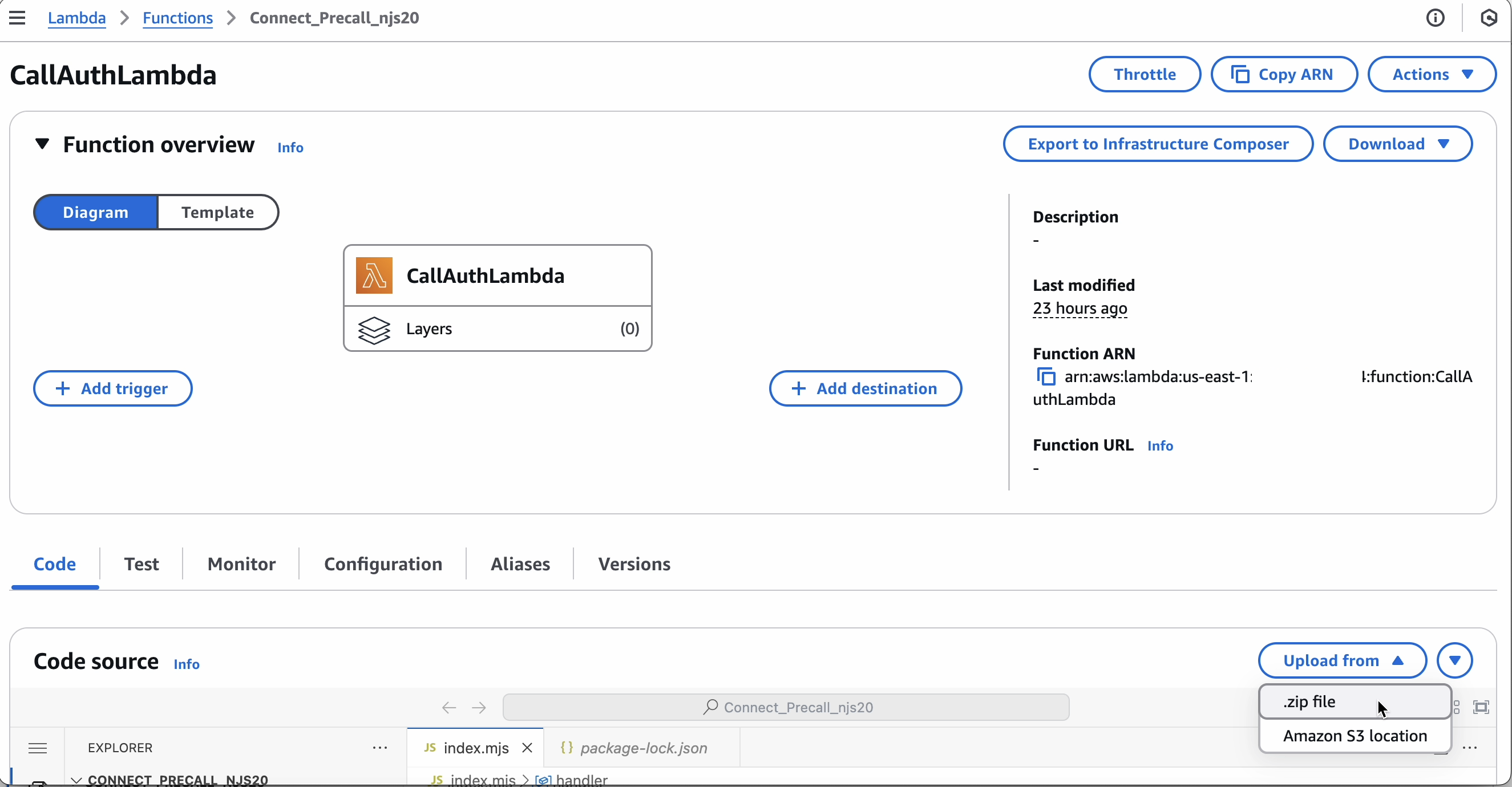
3. Add API Keys to Lambda Environment Variables
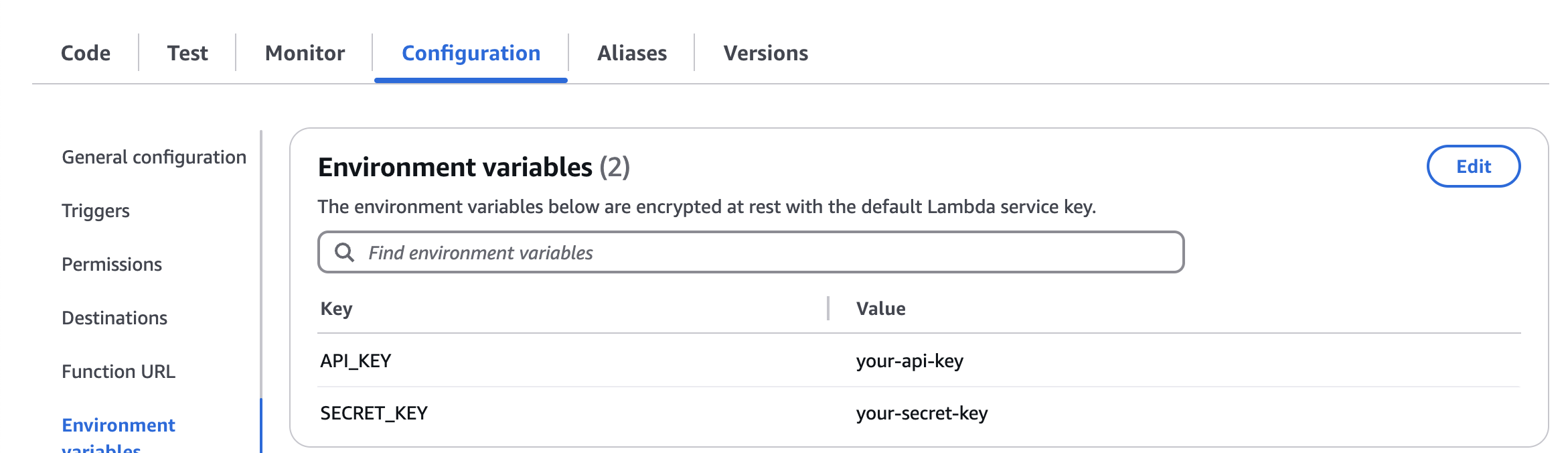
Using the Lambda
Change the a_number and b_number variables in the code to correspond to the payload from your input source.
- This example is being used by Amazon Connect. Connect documentation: doc link
// index.mjs
...
export const handler = async (event) => {
var aNumber = event.Details.ContactData.SystemEndpoint.Address // Phone number making the call.
var bNumber = event.Details.ContactData.CustomerEndpoint.Address // Phone number being called.
...
// index.mjs
...
export const handler = async (event) => {
var a_number = "+15555555555" // Phone number making the call.
var b_number = "+15554444444"
...
- Example Amazon Connect Outbound Whisper Flow
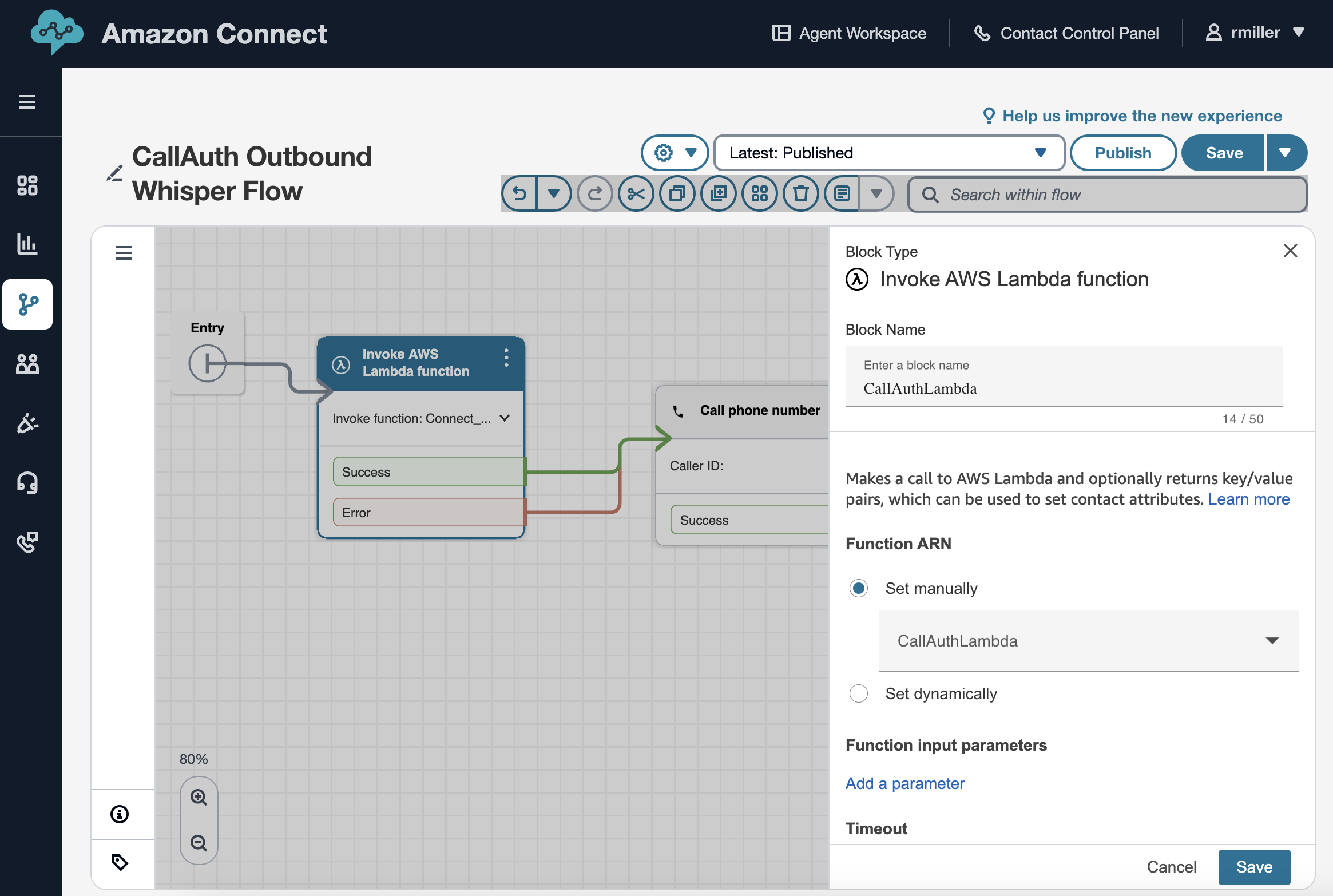
Updated 11 days ago