Affirm Testing & Automation
This guide will cover Affirm Testing and ways to automate it.
Getting Started
How does Affirm work?
First Orion will give a Business three Phone Numbers, one for each of the 3 major U.S. mobile networks. The business needs to call each to build out the Affirm report. This means each Phone Number a Business wants to check, needs to make a phone call to all three numbers (carriers).
Pre-requisites
- Affirm Reporting added to the Business
- Three Affirm Phone Numbers
- Phone Call Platform
- Platform to host automation (AWS, Azure, Localhost, etc)
Making the Calls
To get the most accurate reporting, it is best to make the call look similar to an Agent making a call. The preferable method would be to originate the test calls from the Calling Platform with calling lists, APIs or other technologies.
Automate the Calls
Automate a call just means that a call will be happen at either a certain time or frequency. This can be done with a local script, cloud platform, or on the native call platform (if it's available).
Making the Calls
Here are some options, using specific examples, to initiate making a phone call to Affirm using specific platforms.
Manual Dialing
This is the simplest way to test with Affirm. Agents will need to call all three phone numbers manually from their Phone Dialer.
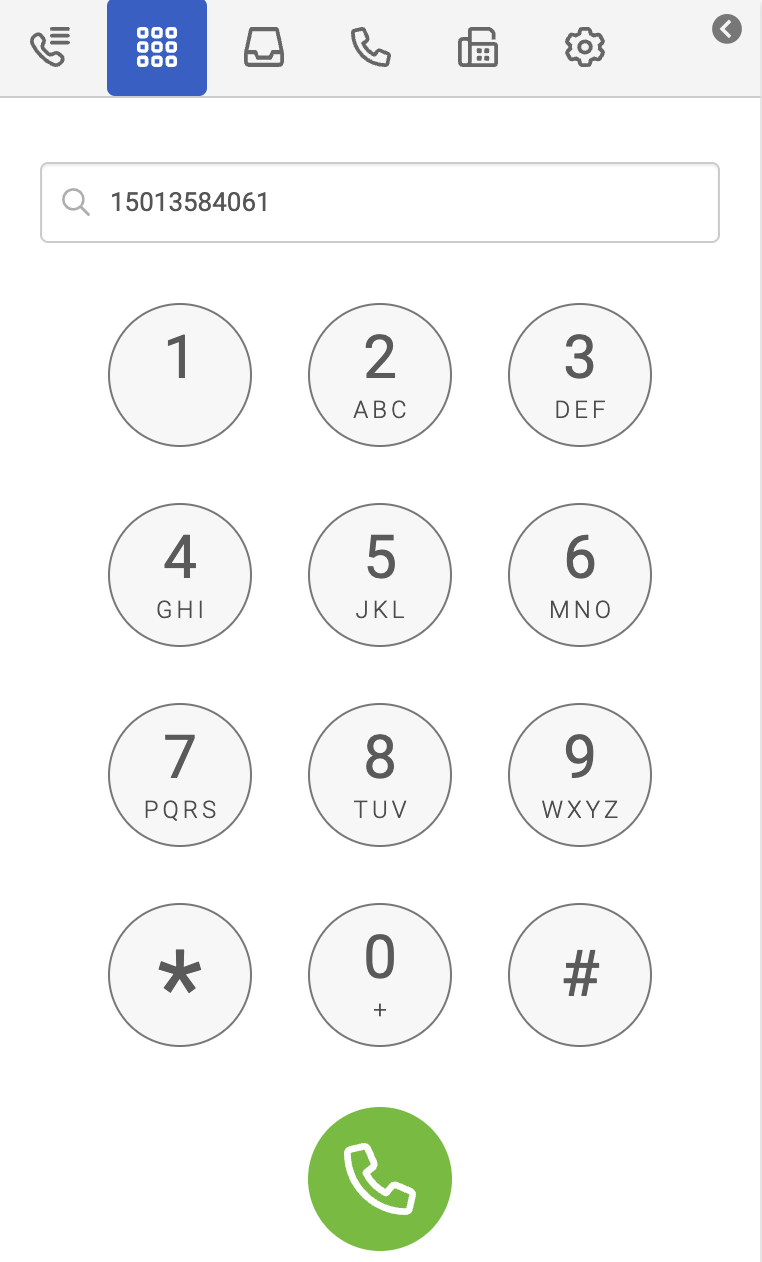
Power Dialing
This is the automated dialing many platforms use to automate outbound phone calls from their Agents, usually setup by a Manager. Affirm test numbers can be inserted into the usual dialing list to automate the test calls (see automation example below).
API Endpoint
Many calling platforms have APIs that let the business start outbound phone calls. This can be used to automate calls.
Twilio used in this example: (See this Twilio Example)
# Python 3.10
# This example uses Twilio's Rest API
### Libraries
import requests
from requests.auth import HTTPBasicAuth
### Authentication
# Find your Account SID and Auth Token at twilio.com/console
# and set the environment variables. See http://twil.io/secure
account_sid = "{TWILIO_ACCOUNT_SID}"
auth_token = "{TWILIO_AUTH_TOKEN}"
### Phone Numbers
data = {
'From': '+15555555555', # Your Twilio phone number
'To': '+15013584061', # The phone number you are calling
'Url': 'http://demo.twilio.com/docs/voice.xml' # URL for TwiML instructions
}
### Create Phone Call
url = f'https://api.twilio.com/2010-04-01/Accounts/{account_sid}/Calls.json'
response = requests.post(url, data=data, auth=HTTPBasicAuth(account_sid, auth_token))
print(response.text)
Native Library
Some calling platforms have native libraries that let the business start outbound phone calls. This can be used to automate calls.
Twilio used in this example: (See this Twilio Example)
# Python 3.10
# This example uses Twilio's Python Library
### Libraries
# Download the helper library from https://www.twilio.com/docs/python/install
import os
from twilio.rest import Client
### Authentication
# Find your Account SID and Auth Token at twilio.com/console
# and set the environment variables. See http://twil.io/secure
account_sid = "{TWILIO_ACCOUNT_SID}"
auth_token = "{TWILIO_AUTH_TOKEN}"
client = Client(account_sid, auth_token)
### Create Phone Call
call = client.calls.create(f
twiml="<Response><Say>Affirm Call!</Say></Response>",
to="+15013584061", ### Phone Numbers
from_="+15555555555",
)
print(call.sid)
Automate Calls with Local Machine
This is as simple as adding the script to a scheduling process.
Steps to automate calls on a local machine
- Use a script similar to the one below. This one will call every phone number in the Affirm Number list from each phone number in Business Numbers list.
- Replace the makephone_call function with a script similar to the one from the _Making the Calls section.
- Run the script. This example will loop and start the process over after the last call.
# Python 3.10
### How this works.
# This script will call every phone number in the Affirm Number list from each
# phone number in Business Numbers list.
#
# Replace the make phone call function with the needed script (See steps to initiate call).
import time
def make_phone_call(affirm_number,business_number):
## Fill this function with the needed script.
return
affirm_numbers = [
"+14155551212",
"+14155551213",
"+14155551214"
]
business_numbers = [
"+15017122661",
"+15017122662",
"+15017122663",
"+15017122664",
"+15017122665",
]
while True:
for business_number in business_numbers:
for affirm_number in affirm_numbers:
print(f"Calling {affirm_number} from {business_number}")
make_phone_call(affirm_number,business_number)
time.sleep(180) # Waits 180 seconds then makes next call.
# Python 3.10
### How this works.
# This script will call every phone number in the Affirm Number list from each
# Twilio phone number in Business Numbers list.
import time
# Download the helper library from https://www.twilio.com/docs/python/install
import os
from twilio.rest import Client
### Authentication
# Find your Account SID and Auth Token at twilio.com/console
# and set the environment variables. See http://twil.io/secure
account_sid = "{TWILIO_ACCOUNT_SID}"
auth_token = "{TWILIO_AUTH_TOKEN}"
client = Client(account_sid, auth_token)
def make_phone_call(affirm_number,business_number):
call = client.calls.create(f
twiml="<Response><Say>Ahoy, World!</Say></Response>",
to=affirm_number, ### Phone Numbers
from_=business_number
)
print(call.sid)
return
affirm_numbers = [
"+14155551212",
"+14155551213",
"+14155551214"
]
business_numbers = [
"+15017122661",
"+15017122662",
"+15017122663",
"+15017122664",
"+15017122665",
]
while True:
for business_number in business_numbers:
for affirm_number in affirm_numbers:
print(f"Calling {affirm_number} from {business_number}")
make_phone_call(affirm_number,business_number)
time.sleep(180) # Waits 180 seconds then makes next call.
Automate Calls with AWS
This example uses AWS to host our automation, but other cloud services should have similar functionality.
Outline
- Lambda Setup
- Create Lambda
- Create an EventBridge Schedule to trigger the Lambda
1. Lambda Setup
Lambda Directory and Code
- Create a local directory with this lambda_function.py file inside. Later, this will be the Lambda zip folder that's uploaded.
- Replace the make_phone_call function with the needed functionality for the platform. (See generic tab for Example)
# Python 3.10
### How this works.
# This Lambda Function will call an Affirm phone number from a Business phone number.
# Both need to be passed into the event with this payload structure.
#
# Replace the make phone call function with the needed script (See steps to initiate call).
from twilio.rest import Client
def lambda_handler(event, context):
calls_to_make = event["calls"]
for call in calls_to_make:
affirm_number=call['affirm_number']
business_number=call['business_number']
print("affirm_number: " + affirm_number)
print("business_number: " + business_number)
print("twilio call id: "+make_phone_call(affirm_number,business_number))
def make_phone_call(affirm_number,business_number):
account_sid = "{TWILIO_ACCOUNT_SID}"
auth_token = "{TWILIO_AUTH_TOKEN}"
client = Client(account_sid, auth_token)
call = client.calls.create(
url="http://demo.twilio.com/docs/voice.xml",
to=affirm_number,
from_=business_number
)
return call.sid
# Python 3.10
### How this works.
# This Lambda Function will call an Affirm phone number from a Business phone number.
# Both need to be passed into the event with this payload structure.
#
# Replace the make phone call function with the needed script (See steps to initiate call).
import json
import boto3
from botocore.vendored import requests
def lambda_handler(event, context):
affirm_number=event['affirm_number']
business_number=event['business_number']
print(f"Calling {affirm_number} from {business_number}")
make_phone_call(affirm_number,business_number)
return {
'statusCode': 200,
'body': json.dumps('Hello from Lambda!')
}
def make_phone_call(affirm_number,business_number):
## Fill this function with the needed script.
return
Lambda Code Libraries
This Lambda example requires the Twilio library to be included in the Lambda directory. Here is a method to include that library.
-
Navigate to the directory containing the lambda_funciton.py file.
-
Open this directory in a terminal and run the following. This should create the necessary library folders.
pip3 install twilio -t ./
pip install requests -t ./
-
Compress/ZIP those folders with the lambda code from inside the folder. This will be uploaded to the Lambda
2. Create the Lambda
- Environment Information
- Function Runtime: Python3.x
- Architecture: x86_64
- Upload Lambda Zip
- This is the compressed folder from above
3. Create a EventBridge Schedule
This Schedule will pass the payload of numbers to call. The phone numbers can be hardcoded in the Lambda for a more simple setup.
- Go to EventBridge and Create a Schedule
- Name the Schedule and give it a description.
- Specify the Schedule Pattern Detail
- Set Occurrence to Recurring schedule
- Set Cron-based schedule for how often you want the call to happen. (See example below)
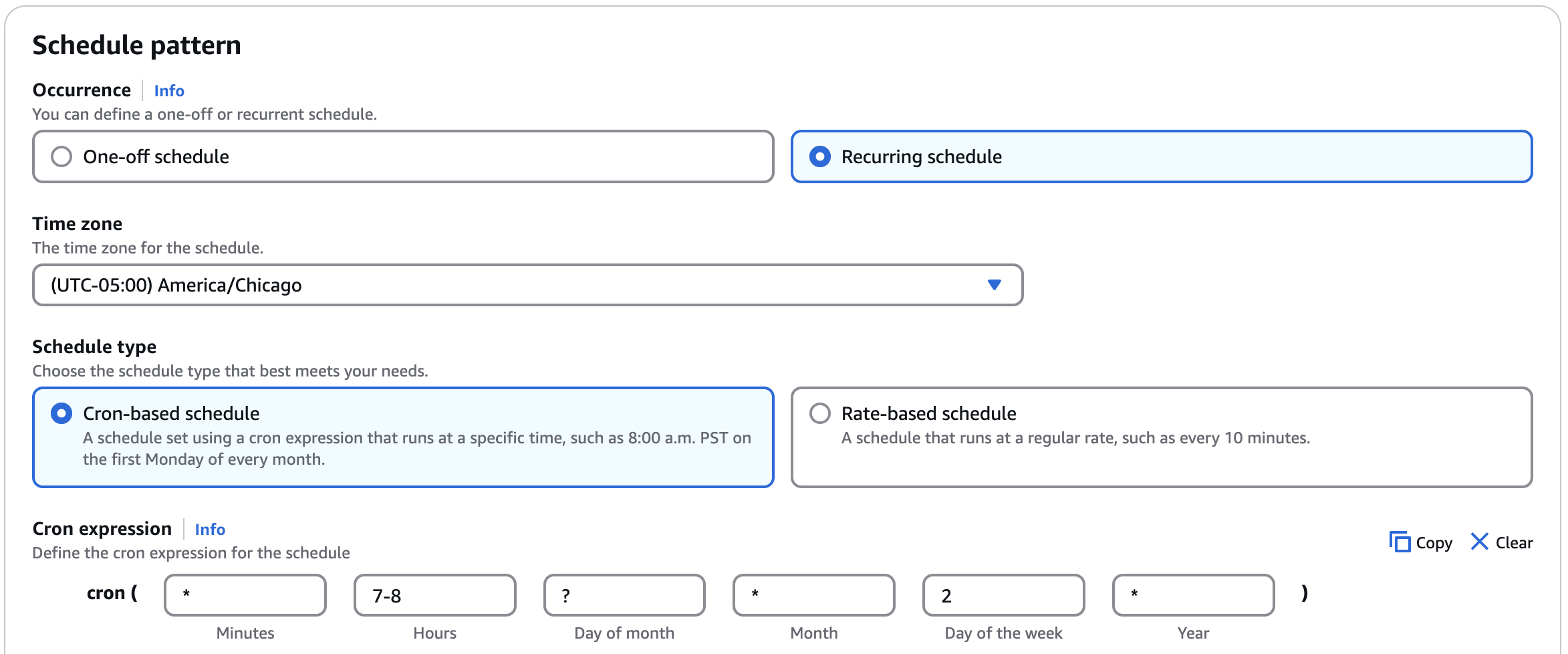
Example: Cron expression for every minute, between 07:00 AM and 08:59 AM, only on Tuesday
- Select AWS Lambda Invoke as the Target.
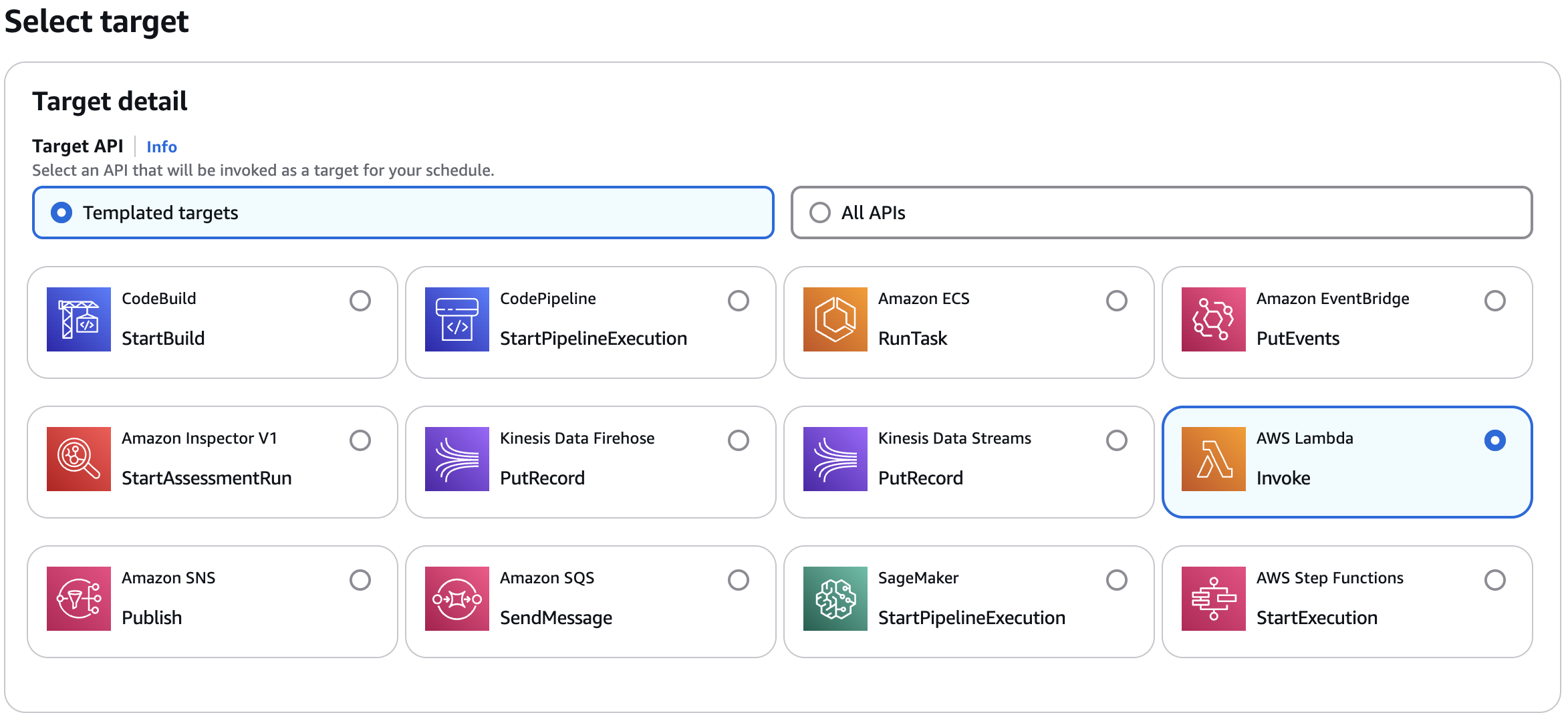
- Set the Lambda under Invoke to the Lambda just created. Add the following payload.
-
{ "calls":[ {"affirm_number":"+12136765030","business_number":"+18669876366"}, {"affirm_number":"+13128485581","business_number":"+18335668036"}, {"affirm_number":"+13039942300","business_number":"+18772071391"} ] }
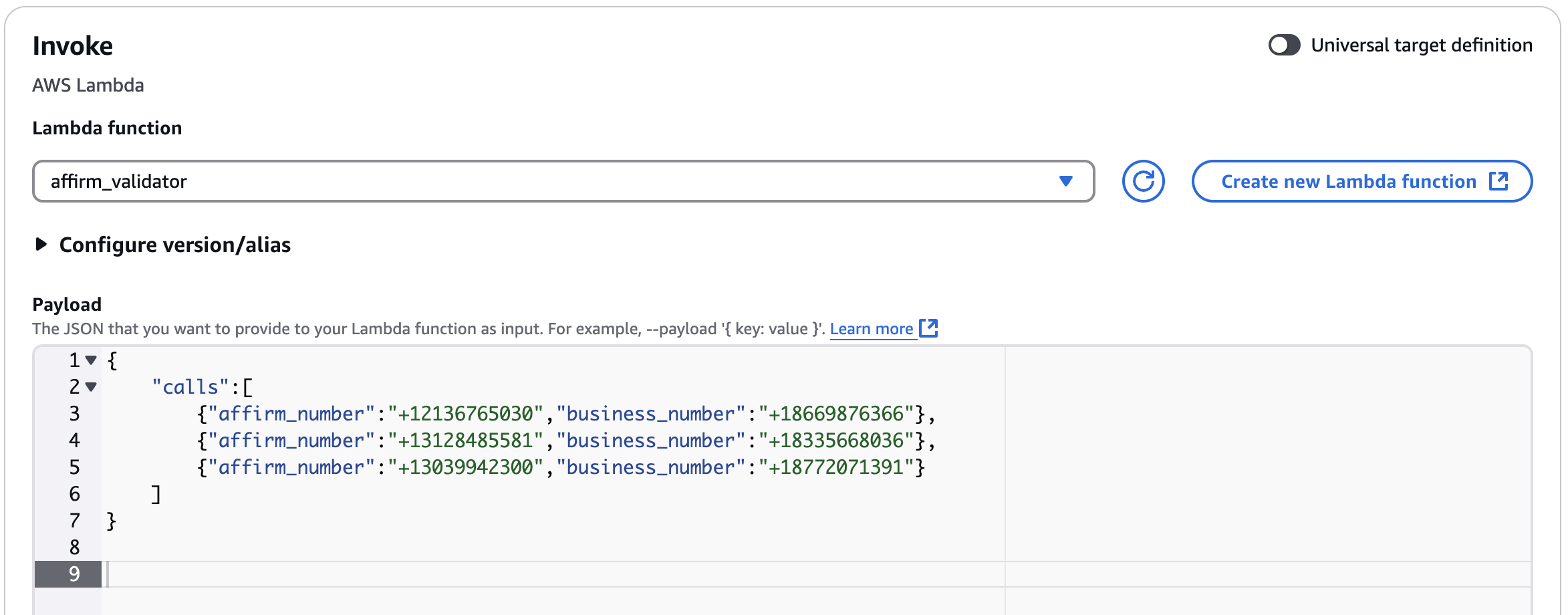
- Review and create the Schedule
- The other Schedule settings can stay as default.
- Review Logs after calls

Example Lambda Logs from code above
Automate Calls with Contact List (Genesys)
This example uses Genesys to show how Affirm numbers can be included in the regular Conact/Lead List.
Outline
- Create a list with Affirm numbers included.
- Add list to Outbound Campaign
- Make Calls
1. Create Contact List
For all work in Genesys, go to the Admin tab.
- Create a CSV with at least the following headers. (See example)
- phone
- type
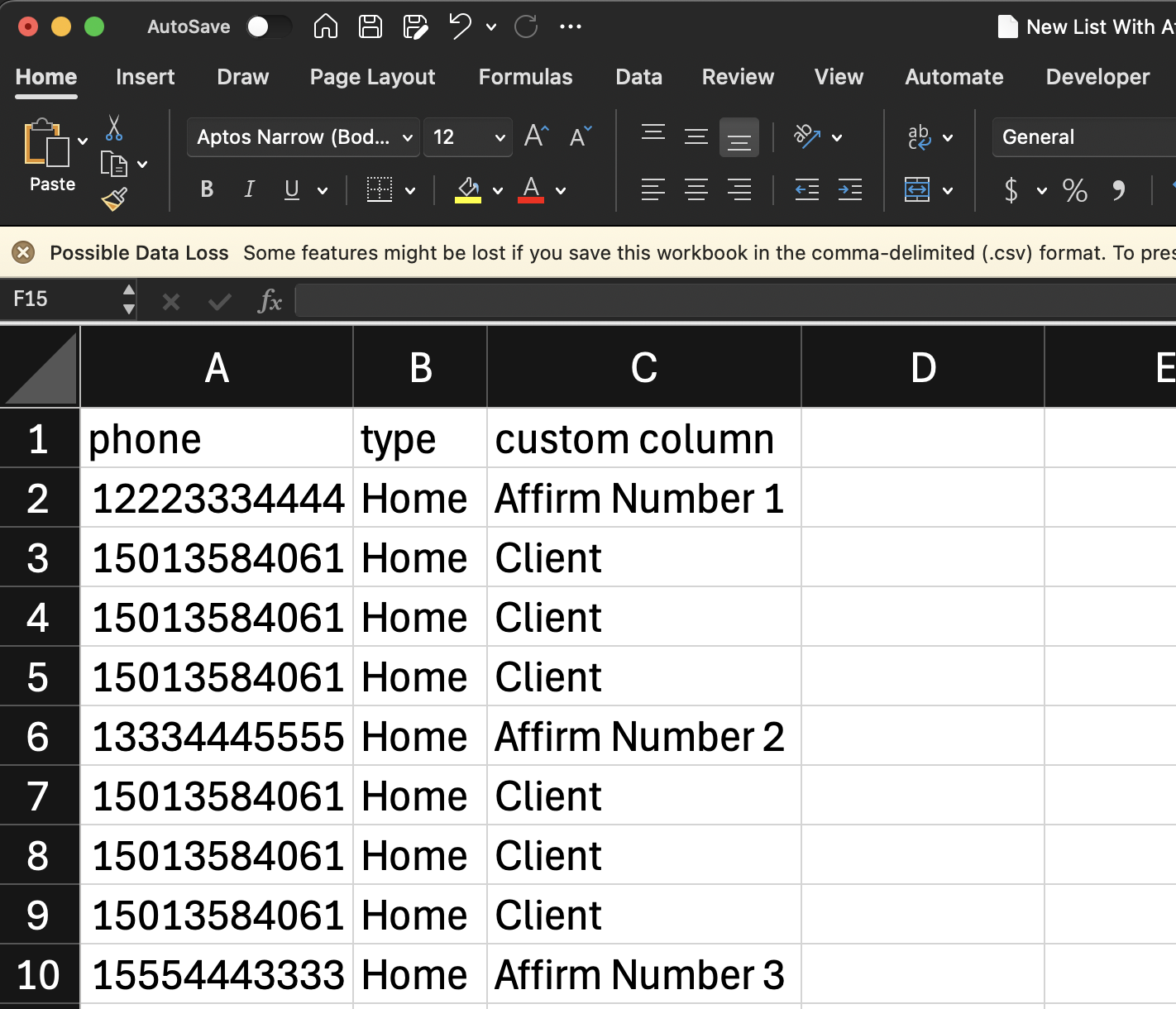
This example shows the three Affirm numbers mixed in with Client numbers
- In the Genesys portal, under the Outbound section, click on List Management
- Click the Create New button under Contact Lists and upload the new list.
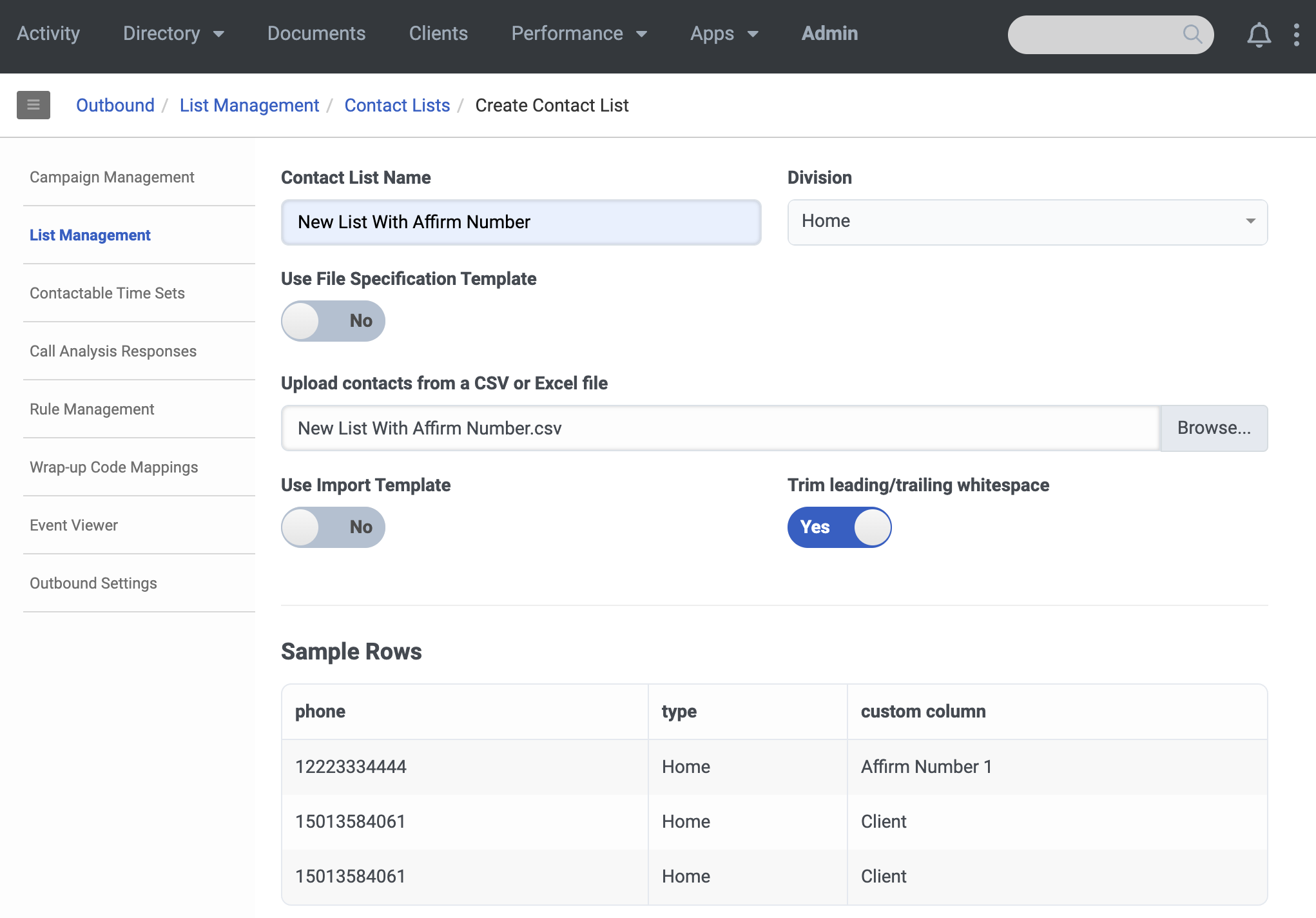
2. Add Contact List to Genesys Campaign
In Genesys go to the Admin tab.
- In the Genesys portal, under the Outbound section, click on Campaign Management
- Click into the desired Campaign
- Under Campaign Options and Contact List, select the New Contact List
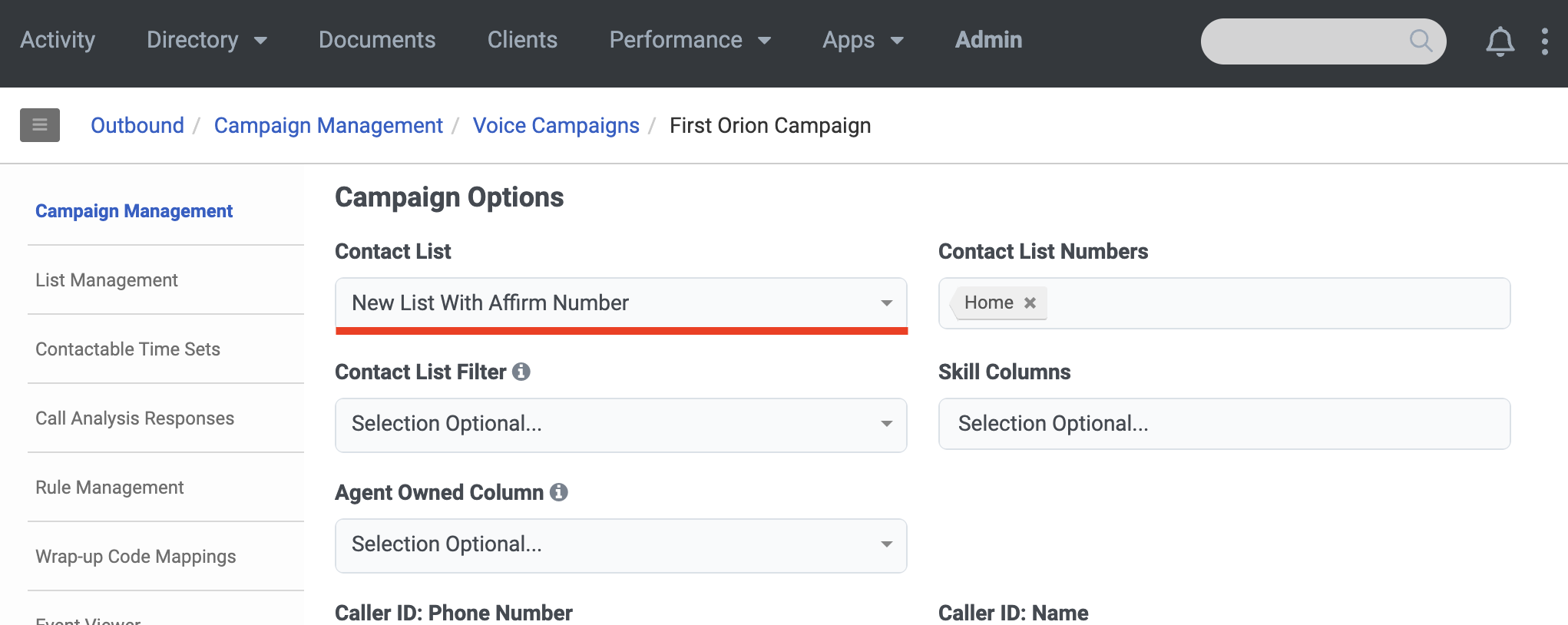
- Save the changes.
- Make calls on the campaign like normal.
Updated 3 months ago