Creating Programs via API
This guide will cover creating different Program types via API.
Pre-requisites
- First Orion Branded Communications agreement
- Access to First Orion Customer Portal
- Vetted and Approved Business
- Understanding of First Orion's Authentication Endpoint
- A Delivery Channel to assign to a Program
- A Phone Number to assign to a Program
- Tool/Service to invoke API Endpoints (cURL examples shown here)
Understanding a Program
Data Hierarchy and Make Up
Programs can be thought of as a container that associates phone numbers to their network behaviour, like Branding.
Each program will have:
- Program: The "container" itself.
- Schedule: Dates for the Branding to be active.
- Delivery Channels: Determines the Programs behaviour.
- Phone Numbers: The desired calling phone numbers for the Program.
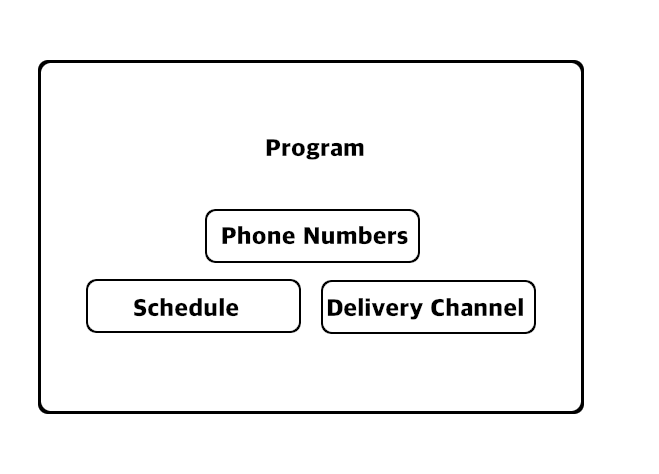
Create a Program
Step overview
- Create a Program (container)
- Create a Delivery Channel (See separate guide for more information)
- Create and Update a Schedule
- Add Phone Numbers
1. Create the Program
Note: A schedule can be included on creation to reduce the amount of API endpoints.
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
[
{
"programName": "Credit Card Fraud Alert",
"active": true,
"callPurposeCode": "CS",
"dailyCallCountEstimateRangeId": 4,
"authenticatedCalls": true
}
]
'
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
[
{
"programName": "Credit Card Fraud Alert",
"active": true,
"callPurposeCode": "CS",
"dailyCallCountEstimateRangeId": 4,
"authenticatedCalls": true,
"schedules": [
{
"startTime": "1637165491",
"endTime": "1767197478"
}
]
}
]
'
2. Create a Delivery Channel
For more information on Delivery Channel types, check out this guide.
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs/programId/delivery-channels/callerName \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
{
"asset": {
"longName": "Good Invest - Fraud Alert",
"shortName": "Fraud Alert"
}
}
'
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs/programId/delivery-channels/callerImage \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
{
"asset": {
"displayNameContentId": "8adb9766-2ad0-453a-8a99-ed2b281e5b06",
"subjectContentId": "e6ada17e-419d-408a-91ad-f060260a5db2",
"imageContentId": "e6ada17e-419d-408a-91ad-f060260a5db2",
"engageEnabled": true,
"informPremiumEnabled": true
}
}
'
3. Create and Update a Schedule
Note: Schedules are in Epoch time.
- An update example is in the second tab.
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs/programId/schedules \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
[
{
"startTime": "1637165491",
"endTime": "1767197478"
}
]
'
curl --request PUT \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/business-units/businessUnitId/programs/programId/schedules \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
[
{
"startTime": "1637165491",
"endTime": "1775658337"
}
]
'
4. Add Phone Numbers
For more information on Phone Number management, check out this guide.
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/phone-numbers \
--header 'Authorization: Token String' \
--header 'content-type: application/json' \
--data '
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "ec0ce06a-3739-45f5-a804-5468e60a843f",
"programId": "df567c99-9354-47e5-b30f-88ee7cbfeab4",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
'
Updated about 2 months ago