Phone Number Management
This will cover how to assign, update and delete phone numbers in a Business with First Orion's API.
Pre-requisites
- First Orion Branded Communications agreement
- Access to First Orion Customer Portal
- Vetted and Approved Business
- Understanding of First Orion's Authentication
- Phone Numbers to Manage
- Tool/Service to invoke the Phone Number Endpoints (cURL examples shown here)
Understanding a Phone Numbers Assignment in First Orion
Data Hierarchy
Phone Numbers are assigned at a few different levels. All phone numbers that are created in First Orion are automatically in a Business Level. This will associate the phone numbers with the business for purposes of tagging and management.
Phone Numbers assigned at the Business Unit Level are essentially being sorted and prepped for the Program Level.
Phone Numbers assigned at the Program Level are actively tied to Branding or Blocking. This means they will behave and display as desired, unless the Programs Expiration Date has passed.
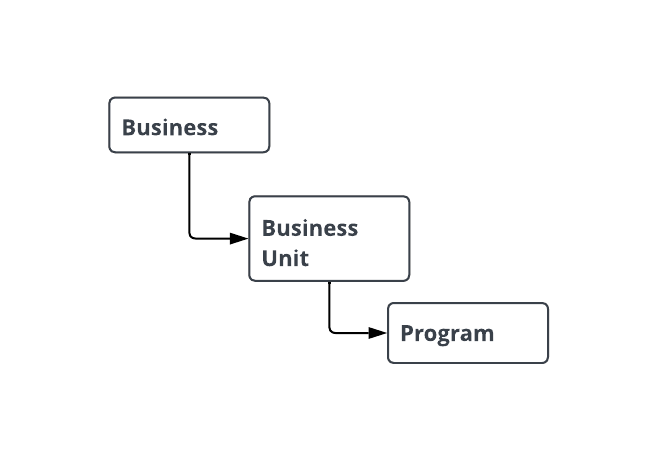
Allocated Numbers
When a phone number is created in First Orion, it can only be branding in one Business at a time. So if Business Alpha uploads a Phone Number for Branding, Business Bravo will get an error saying, "ALLOCATED_NUMBER", if they try to Create the same Phone Number for Branding.
Here is an example of an Allocated Number.
{
"body": {
"phoneNumbers": [],
"rejectedPhoneNumbers": [
{
"phoneNumber": "+15012149020",
"callPurposeCode": "CS",
"rejectionReason": "ALLOCATED_NUMBER",
"allocatedBusinessId": "cb5ef552-ba40-49ee-a722-57d820f48cd3",
"allocatedPhoneNumberId": "f790b110-41e4-4bac-bcf7-8b117533e010"
}
]
},
"error": null
}
Creating a New Phone Number
The following steps detail the process of Creating a new Phone Number using the Create Business Phone Number Endpoint.
Step 1 - Get Token
Check out the Authentication Endpoints to get a token.
Step 2 - Gather Relationship IDs
Phone number assignment is based around the related Relationship IDs. Creating and Updating a phone number can use the same Body format with various Relationship IDs to determine phone number assignment.
Using First Orion Portal
-
Login
-
Notice the Business ID behind "business/" in the URL. Save that for later.
-
Scroll down and click a Business Unit.
-
Notice the Business Unit ID behind "businessUnit/" in the URL. Save that for later.
-
While at the Business Unit Level, scroll down and click a Program (Not Pictured). Notice the Program ID on the bottom left. Save that for later.
Using First Orion API
- Use the List Business Endpoint to pull your businesses information. Save the Business ID in the response (See response to endpoint in documentation).
- Use the List Business Units Endpoint to pull the business units. Save the desired Business Unit ID.
- Use the List Programs Endpoint to pull the programs. Save the desired Program ID.
Step 3 - Build and Submit the Request
Build Request Body
- Call Purpose Code - This is used by carriers. Find the full list of codes here.
- Authenticated Calls - If you are using standard Inform, this will stay false. If you are using Authenticated Call Branding, this will be true.
- Relationships - Determines where to assign the Phone Number.
- IDs (All three) - Associate the Phone Number to a Brand (These are the three IDs saved earlier).
- Caller Name - This is required when assigning the phone number to a Branding Program.
- Phone Number - The phone number to Create in E.164 format.
Submit the Request
Check the second tab for a cURL example.
- Set the request method to POST.
- Add the business Authorization Token to the header.
- Fill in the request body data.
- Submit the request.
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "ec0ce06a-3739-45f5-a804-5468e60a843f",
"programId": "df567c99-9354-47e5-b30f-88ee7cbfeab4",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/719d140e-fd6c-4d59-9241-3dee71b38320/phone-numbers \
--header 'Authorization: token string' \
--header 'Ccontent-Type: application/json' \
--data '
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "ec0ce06a-3739-45f5-a804-5468e60a843f",
"programId": "df567c99-9354-47e5-b30f-88ee7cbfeab4",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
'
{
"body": {
"phoneNumbers": [
{
"phoneNumberId": "0dd9a02c-03f9-49d5-aa83-7e9498fbe74f",
"phoneNumber": "+12125554200",
"lastStatusChangeTimestamp": "1639711517",
"submittedTimestamp": "1639711517",
"status": "PENDING",
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "ec0ce06a-3739-45f5-a804-5468e60a843f",
"programId": "df567c99-9354-47e5-b30f-88ee7cbfeab4",
"callerName": true
}
}
],
"rejectedPhoneNumbers": []
},
"error": null
}
Updating an Existing Phone Number
The following steps detail the process of Updating a Phone Numbers relationship to update the Branding/behaviour.
Step 1 - Get Token
Check out the Authentication Endpoints to get a token.
Step 2 - Build Your Request Body
Check Step 2 - Gather Relationship IDs under the Creating a New Phone Number section to learn how to get other relationship IDs.
This Step will be broken down into the following sections:
- Assign to new Program and Business Unit
- Assign to new Business Unit Only
- Remove from Program
- Remove from Business Unit.
Assign to New Program and Business Unit
When updating to phone number to a different Program with this endpoint and method, the Program can be in the current Business Unit or assigned to a new Business Unit and Program in the same request.
Notice:
- The first request body has a new Program and uses the original Business Unit ID.
- The second request body has a new Program and new Business Unit ID.
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "ec0ce06a-3739-45f5-a804-5468e60a843f",
"programId": "6d3580a0-f667-425e-84b5-e7df13cc3e1b",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "qbymn4fd-dgy9-nfb6-4ham-7ohen2plvkmm",
"programId": "8jj2mqcf-vf7m-wjob-g5gc-92478co69n11",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
Assign to New Business Unit Only
This will only associate the Phone Number up to the Business Unit level. This will result in no Branding.
Notice:
- It has the Program Details removed
- The new Business Unit ID
[
{
"phoneNumber": "+12125554200",
"callPurposeCode": "CS",
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "n2kurzi0-cqmo-e533-vdqh-4emaww567ax2"
}
}
]
Remove from Program
This will un-associate the Phone Number from a Program.
Notice:
- This is the same exact request as the Assign to new Business Unit Only section. This is an example of different use cases using the same endpoint and information.
[
{
"phoneNumber": "+12125554200",
"callPurposeCode": "CS",
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "n2kurzi0-cqmo-e533-vdqh-4emaww567ax2"
}
}
]
Remove from Business Unit
This will un-associate the Phone Number from a Business Unit, so it will only be assigned to the Business.
Notice:
- The Business ID is still needed in the request body.
[
{
"callPurposeCode": "CS",
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320"
},
"phoneNumber": "+12125554200"
}
]
Step 3 - Submit the Request
Build out the request by
- Setting the Business ID in the URL
- Setting the Authorization Token in the Headers
- Fill out the Request Body according to use case using the Relationship IDs from the previous steps.
curl --request PUT \
--url https://api.firstorion.com/exchange/v1/businesses/719d140e-fd6c-4d59-9241-3dee71b38320/phone-numbers \
--header 'Authorization: token-string' \
--header 'accept: application/json' \
--data '
[
{
"callPurposeCode": "CS",
"authenticatedCalls": false,
"relationships": {
"businessId": "719d140e-fd6c-4d59-9241-3dee71b38320",
"businessUnitId": "qbymn4fd-dgy9-nfb6-4ham-7ohen2plvkmm",
"programId": "8jj2mqcf-vf7m-wjob-g5gc-92478co69n11",
"callerName": true
},
"phoneNumber": "+12125554200"
}
]
'
Delete Phone Numbers
Step 1 - Get Token
Check out the Authentication Endpoints to get a token.
Step 2 - Select Delete Method and Submit the Request
Deleting will remove the Phone Number from the business completely. This is useful for cleaning out a phone number inventory in the First Orion platform.
Endpoints:
- Delete All Phone Numbers - DO NOT USE. This is only useful for deleting every phone number registered to the business.
curl --request DELETE \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/phone-numbers \
--header 'accept: application/json'
- Delete Phone Number - NOT RECOMMENDED TO USE. This is similar to the first endpoint. If the Phone Number ID isn't added to the URL, ALL Phone Numbers under that Business could be deleted.
curl --request DELETE \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/phone-numbers/x7ssz559-62a8-v3d2-p7xa-0wafb6ljzei1 \
--header 'accept: application/json'
- Delete Phone Numbers - RECOMMENDED. This endpoint requires a request body with a list of Phone Numbers or Phone Number IDs. This makes it the most convenient and safest way to Delete Phone Numbers from a Business. Notice this is a POST method with a new URL instead of DELETE.
- Notice the two different requests. Both requests will delete the same phone numbers.
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/phone-numbers/DELETE \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"phoneNumbers": [
"+12125554200"
]
}
'
curl --request POST \
--url https://api.firstorion.com/exchange/v1/businesses/businessId/phone-numbers/DELETE \
--header 'accept: application/json' \
--header 'content-type: application/json' \
--data '
{
"phoneNumberIds": [
"0dd9a02c-03f9-49d5-aa83-7e9498fbe74f"
]
}
'
Updated 2 months ago